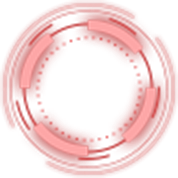
地图图层加载
本章介绍地图组件中各种图层的加载。
基础用法
<template>
<div style="position: relative; width: 100%; height: 500px; overflow: hidden">
<minemap />
</div>
</template>
<script setup>
import minemap from './components/index.vue';
</script>
<style lang="scss" scoped></style>
加载普通点位
加载普通图标到地图上
point-normal-list
是传入的普通点位列表,title
代表鼠标移入需要显示的名称,icon
为需要展示的图标
注意
加载到地图上的 icon
首先需要在 staticUtil
文件中引入载入地图中
move-call-back
是传入的鼠标移动触发的方法需要配合 add-event-layer
一起使用
add-event-layer
是传入的需要添加事件的具体图层
@createNormalPopup
是地图组件中创建普通信息弹框后抛出的事件,配合鼠标移入时展示信息使用
<template>
<div style="position: relative; width: 100%; height: 500px; overflow: hidden">
<minemap
map-dom-id="normal"
:point-normal-list="pointNormalList"
:add-event-layer="addEventLayer"
:move-call-back="mapMoveCallBack"
@createNormalPopup="createNormalPopup"
/>
</div>
</template>
<script setup>
import minemap from './components/index.vue';
const pointNormalList = [
{
id: 1,
title: '江苏省海安高级中学',
lng: 120.468851,
lat: 32.555493,
icon: 'school', //必须为staticUtil中加载到地图中的图片
},
{
id: 2,
orgId: 2000007,
title: '王府大酒店',
lng: 120.491374,
lat: 32.538508,
icon: 'hotel',
},
{
id: 3,
title: '智达教育(海安校区)',
lng: 120.471218,
lat: 32.537631,
icon: 'school',
},
];
const addEventLayer = ['normalLayer'];
let mapNormalpopup = null;
let mapPopupSetContent = null;
const createNormalPopup = (normalPopup, popupSetContent) => {
mapNormalpopup = normalPopup;
mapPopupSetContent = popupSetContent;
};
const mapMoveCallBack = (point, popupOption = { isShow: true }) => {
// 鼠标移入相关点位就默认显示离开就隐藏
if (popupOption.isShow) {
mapPopupSetContent(mapNormalpopup, point);
} else {
mapPopupSetContent(mapNormalpopup, { lng: 0, lat: 0 });
}
};
</script>
<style lang="scss" scoped></style>
加载区域范围的点位
地图组件内部使用 message-pupup.vue
组件来加载点位信息弹框
pointBuildList
是传入的标记范围的点位列表,title
是要展示的信息的头部
icon
是点位颜色包含 redPoint
、bluePoint
和 greenPoint
三种已经内置到地图组件中
getMessagePopupBg
是信息弹框背景色一般来说和 icon
的颜色保持一致
可以通过点位的某个字段来规定颜色,同样已经内置到地图组件中。共三种 red
、blue
和 green
<template>
<div style="position: relative; width: 100%; height: 500px; overflow: hidden">
<minemap
map-dom-id="building"
:point-build-list="pointBuildList"
:get-message-popup-bg="getMessagePopupBg"
>
<template #messagePopup="{ point }">
<div class="mt-sm message-popup" v-if="point.buildingNumber">
<div>
<span class="message-title">楼栋:</span>
<span class="message-num">{{ point.buildingNumber }}</span>
</div>
<div>
<span class="message-title">房屋:</span>
<span class="message-num">{{ point.houseNumber }}</span>
</div>
</div>
</template>
</minemap>
</div>
</template>
<script setup>
import minemap from './components/index.vue';
const pointBuildList = [
{
id: 1,
title: '海安市政府',
lng: 120.467735,
lat: 32.532712,
icon: 'redPoint',
},
{
id: 2,
title: '书香园小区',
lng: 120.468946,
lat: 32.537649,
icon: 'bluePoint',
buildingNumber: 20,
houseNumber: 1440,
},
{
id: 3,
title: '江苏省海安高级中学',
lng: 120.468851,
lat: 32.555493,
icon: 'greenPoint',
},
{
id: 4,
title: '王府大酒店',
lng: 120.491374,
lat: 32.538508,
icon: 'redPoint',
},
{
id: 5,
title: '智达教育(海安校区)',
lng: 120.471218,
lat: 32.537631,
icon: 'greenPoint',
},
{
id: 7,
title: '123',
lng: 120.472562307338,
lat: 32.5334267105474,
icon: 'bluePoint',
},
];
const getMessagePopupBg = (point) => {
return point.icon === 'redPoint' ? 'red' : point.icon === 'greenPoint' ? 'green' : 'blue';
};
</script>
<style lang="scss" scoped>
.message-popup {
display: flex;
justify-content: space-around;
.message-title {
color: rgb(255 255 255 / 80%);
}
.message-num {
font-weight: 900;
color: #fff;
}
}
</style>
加载地图围栏
map2D3D
为地图俯视角度,当为 2D
时展示的是 edit,当为 3D
时展示的是 fence
map-edit-data
是传入的加载区域范围的点位列表,geometry
是需要展示的范围的坐标区域,type
是区域类型默认为 Feature
properties
为 2D
模式时区域范围的边框颜色,填充色,可否编辑等,一般来说颜色与 fenceImgUrl
保持一致
fenceImgUrl
为 3D
模式时围栏的颜色取值
<template>
<div style="position: relative; width: 100%; height: 500px; overflow: hidden">
<minemap map-dom-id="fence" :map2D3D="map2D3D" :map-edit-data="mapEditData">
<template #messagePopup="{ point }">
<div class="mt-sm message-popup" v-if="point.buildingNumber">
<div>
<span class="message-title">楼栋:</span>
<span class="message-num">{{ point.buildingNumber }}</span>
</div>
<div>
<span class="message-title">房屋:</span>
<span class="message-num">{{ point.houseNumber }}</span>
</div>
</div>
</template>
</minemap>
<Button type="primary" style="position: absolute; top: 10px; right: 10px" @click="mapChange">
转换2D3D
</Button>
</div>
</template>
<script setup>
import minemap from './components/index.vue';
import redFence from '/images/map/minemap/red-fence.png';
import greenFence from '/images/map/minemap/green-fence.png';
import blueFence from '/images/map/minemap/blue-fence.png';
import { ref } from 'vue';
const map2D3D = ref('3D');
const mapChange = () => {
map2D3D.value = map2D3D.value === '2D' ? '3D' : '2D';
};
const mapEditData = [
{
id: 1,
type: 'Feature',
properties: {
lineColor: '#F61C35',
fillColor: '#F61C35',
fillOpacity: 0.2,
fillOutlineColor: '#F61C35',
fillOutlineWidth: 2,
isLock: true,
custom_style: 'true',
},
geometry: {
type: 'Polygon',
coordinates: [
[
[120.465828, 32.533069],
[120.468515, 32.534381],
[120.46998, 32.532282],
[120.467324, 32.530908],
[120.465828, 32.533069],
],
],
},
fenceImgUrl: redFence,
},
{
id: 2,
type: 'Feature',
properties: {},
geometry: {
type: 'Polygon',
coordinates: [
[
[120.466307, 32.537685],
[120.469146, 32.539252],
[120.469523, 32.53875],
[120.469523, 32.53875],
[120.471136, 32.538194],
[120.467354, 32.536174],
[120.466307, 32.537685],
],
],
},
fenceImgUrl: greenFence,
},
{
id: 3,
type: 'Feature',
properties: {},
geometry: {
type: 'Polygon',
coordinates: [
[
[120.46706, 32.556076],
[120.469683, 32.55657],
[120.4713, 32.55479],
[120.467761, 32.554341],
[120.46706, 32.556076],
],
],
},
fenceImgUrl: blueFence,
},
{
id: 4,
type: 'Feature',
properties: {},
geometry: {
type: 'Polygon',
coordinates: [
[
[120.490838, 32.538595],
[120.491557, 32.538888],
[120.491611, 32.538439],
[120.491103, 32.538237],
[120.490838, 32.538595],
],
],
},
fenceImgUrl: blueFence,
},
{
id: 5,
type: 'Feature',
properties: {},
geometry: {
type: 'Polygon',
coordinates: [
[
[120.47087, 32.537774],
[120.471112, 32.537877],
[120.471413, 32.537302],
[120.471413, 32.537302],
[120.47087, 32.537774],
],
],
},
fenceImgUrl: blueFence,
},
{
id: 7,
type: 'Feature',
properties: {
lineColor: '#F61C35',
fillColor: '#F61C35',
fillOpacity: 0.2,
fillOutlineColor: '#F61C35',
fillOutlineWidth: 2,
isLock: true,
custom_style: 'true',
},
geometry: {
coordinates: [
[
[120.46610539854002, 32.53577955594519],
[120.46610539854002, 32.53107386514961],
[120.47901921613597, 32.53107386514961],
[120.47901921613597, 32.53577955594519],
[120.46610539854002, 32.53577955594519],
],
],
type: 'Polygon',
},
fenceImgUrl: redFence,
},
];
</script>
<style lang="scss" scoped>
.message-popup {
display: flex;
justify-content: space-around;
.message-title {
color: rgb(255 255 255 / 80%);
}
.message-num {
font-weight: 900;
color: #fff;
}
}
</style>
加载报警点位
地图组件内部使用 warning-popup.vue
组件来加载点位报警信息弹框
alarm-point-list
为报警点位列表,messageType
是用来区分人脸和车辆报警信息
@closeWarningPopup
为关闭报警后所需要处理的方法
<template>
<div style="position: relative; width: 100%; height: 500px; overflow: hidden">
<minemap
map-dom-id="alarm"
:alarm-point-list="alarmPointList"
@closeWarningPopup="closeWarningPopup"
/>
</div>
</template>
<script setup>
import { ref } from 'vue';
import minemap from './components/index.vue';
const alarmPointList = ref([
{
lnglat: [120.456101, 32.53736],
id: '1',
title: `一级`,
similarity: 85.5,
alarmLevel: '一级',
name: '陶尚华',
taskLibName: '人脸测试布控库001',
alarmTime: '2023-11-23 09:24:09',
deviceName: 'B_QN1077延安路东南(结构化2)_(一期)',
alarmPicUrl: '/images/map/minemap/default-photo.png',
taskPicUrl: '/images/map/minemap/default-photo.png',
messageType: 'faceAlarms',
},
]);
// 关闭报警弹框
const closeWarningPopup = (pointId) => {
const index = alarmPointList.value.findIndex((point) => point.id === pointId);
if (index !== -1) {
alarmPointList.value.splice(index, 1);
}
};
</script>
<style lang="scss" scoped></style>